I'm trying to port a tutorial from http://open.gl/drawing to Java using JOGL
I'm able to get output, but I'm receiving an unexpected output.
What is expected:
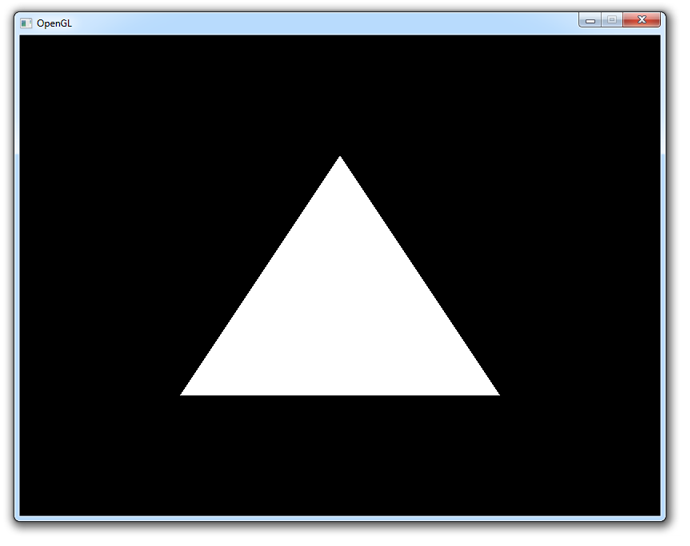
And my output:

It seems that the triangle is not fully facing the camera, but the tutorial does not cover translation or rotation.
GLSL program compilation and linking:
float[] verts = //Our triangle as a set of 2d coordinates
{
0.0f, 0.5f, //vertex 1, top at 0x, -0.5y
0.5f, -0.5f //vertex 2, right at 0.5x, -0.5y
-0.5f, -0.5f //vertex 3, left at -0.5x, -0.5y
};
IntBuffer vao = Buffers.newDirectIntBuffer(1);
gl.glGenVertexArrays(1, vao);
gl.glBindVertexArray(vao.get(0));
IntBuffer vbo = Buffers.newDirectIntBuffer(1);
FloatBuffer vertBuffer = Buffers.newDirectFloatBuffer(verts);
gl.glGenBuffers(1, vbo);
gl.glBindBuffer(GL3.GL_ARRAY_BUFFER, vbo.get(0));
gl.glBufferData(GL3.GL_ARRAY_BUFFER, vertBuffer.limit() * Buffers.SIZEOF_FLOAT, vertBuffer, GL3.GL_STATIC_DRAW);
int vertexShader = gl.glCreateShader(GL3.GL_VERTEX_SHADER);
gl.glShaderSource(vertexShader, 1, vertProgram, null);
gl.glCompileShader(vertexShader);
int fragmentShader = gl.glCreateShader(GL3.GL_FRAGMENT_SHADER);
gl.glShaderSource(fragmentShader, 1, fragProgram, null);
gl.glCompileShader(fragmentShader);
program = gl.glCreateProgram();
gl.glAttachShader(program, vertexShader);
gl.glAttachShader(program, fragmentShader);
gl.glBindFragDataLocation(program, 0, "outColor");
gl.glLinkProgram(program);
gl.glUseProgram(program);
int positionAttribute = gl.glGetAttribLocation(program, "position");
gl.glEnableVertexAttribArray(positionAttribute);
gl.glVertexAttribPointer(positionAttribute, 2, GL.GL_FLOAT, false, 0, 0L);
The display method:
gl.glClearColor(0f, 0f, 0f, 1f);
gl.glClear(GL3.GL_COLOR_BUFFER_BIT);
gl.glDrawArrays(GL.GL_TRIANGLES, 0, 3);
The vertex shader:
#version 330
in vec2 position;
void main()
{
gl_Position = vec4(position, 0.0, 1.0);
}
The fragment shader:
#version 330
out vec4 outColor;
void main()
{
outColor = vec4(1.0, 1.0, 1.0, 1.0);
}